Tailwindcss website defines it as:
A utility-first CSS framework for rapidly building custom designs.
It is a low-level CSS framework that does not provide the ready-to-use, pre-designed components which you normally get with CSS frameworks like Bootstrap and Foundation and the like.
Here is a gist of how it works. Say you want to make a button with a blue background, rounded corners and drop shadow and have the background colour change on mouseover. It will look something like this:
<button class="bg-blue-700 hover:bg-blue-500 rounded shadow-md text-white px-6 py-1">Click</button>
Let get into what this code snippet does. All the styling is done via a bunch of classes.
bg-blue-700
adds background “bg” color #2b6cb0 and when you add hover:
infront of any class, it adds a mouse over. Here the background color changes to #4299e1 on mouse over. By default tailwindcss has many color options available for background, which follows a similar bg-[color] format.
rounded
adds round corners, or border-radius. Specifically it adds a border-radius of 0.25rem. There are other default classes like rounded-sm, rounded-lg, etc which add different size of border radius.
shadow-md
adds a medium box-shadow. Box shadow also has different options available by default like shadow, shadow-md, shadow-lg, etc.
text-white
sets the color to white. By default tailwindcss has many color options available for text as well, which follows a similar text-[color] format.
There are many padding options available. Here I have used px-6
and py-1
. px- adds padding on the x axis, which means it sets padding for left and right. px-6 add padding-left and padding-right of 1.5rem. Similarly, py- adds padding on the y axis.
Margin follows a similar format, where you can use mx-0 to set margin left and right to zero. You can also set padding and margin to specific sides as well, by adding pt- for padding to, or ml- for margin left and similar. Margin has a nagative value option as well, to add a -0.25rem margin you add the class -m-1
.
It will all make sense when you start coding.
Before we get started, here is what we are going to build.
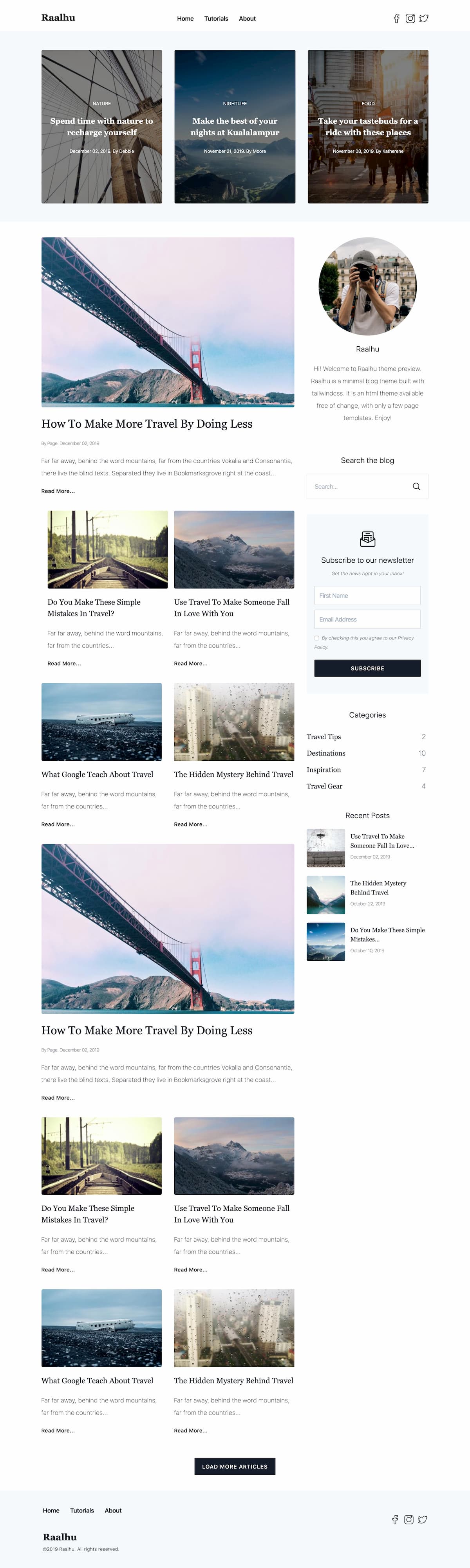
Final Blog Layout
Lets get started and build our theme.
Note: I am using npm to install tailwind, you can also use the CDN version of tailwindcss by adding the blow code to your page header.
<link href="https://unpkg.com/tailwindcss@^1.0/dist/tailwind.min.css" rel="stylesheet">
Read about tailwind installation here.
Header
For the header, I want to have three columns for logo, navigation and socialmedia icons.
<header class="max-w-5xl mx-auto pt-5">
<div class="flex flex-wrap -mx-2 overflow-hidden px-5 lg:px-0">
<div class="my-2 px-2 w-full overflow-hidden md:w-1/6 lg:w-1/3 xl:w-1/3 text-center md:text-left">
<h1 class="font-bold text-2xl font-serif">Raalhu</h1>
</div>
<nav class="my-2 px-2 w-full overflow-hidden md:w-3/6 lg:w-1/3 xl:w-1/3 text-center md:text-left">
<ul>
<li class="inline-block"><a class="block font-semibold px-3" href="">Home</a></li>
...
</ul>
</nav>
<div class="my-2 px-2 w-full overflow-hidden md:w-2/6 lg:w-1/3 xl:w-1/3 text-center md:text-right">
<a href="" title="Facebook" class="inline-block w-6 mr-2">
<svg class="fill-current" viewBox="-110 1 511 512" xmlns="http://www.w3.org/2000/svg">...</svg>
</a>
...
</div>
</div>
</header>
That is the code for the header. A fully responsive three column layout. I used tailwindgrids.com to generate the grid, just because it makes it easier. You can see that I have embedded SVG icons inline with HTML, that is how I normally do it, you can choose to use an icon font or any other method.
I have ommited repeated code and replaced it with … in the snippet above to make it easy to read, and will be repeating the same practice as we go along and will be including full code at the end.
Featured
Again, a three column layout with full image background and text overlay.
<div class="bg-gray-100">
<!-- featured -->
<div class="max-w-5xl mx-auto py-10">
<ul class="flex flex-wrap -mx-2 overflow-hidden">
<li class="my-2 px-2 w-full overflow-hidden md:w-1/3 lg:w-1/3 xl:w-1/3">
<a href="">
<div class="mx-2 flex items-center justify-center bg-gray-300 bg-cover bg-center relative rounded-lg overflow-hidden" style="height:400px; background-image:url(http://lorempixel.com/300/400/nature/)">
<div class="absolute w-full h-full bg-black z-10 opacity-50"></div>
<div class="relative z-20 text-center p-5">
<span class="inline-block text-white uppercase text-xs tracking-wide">Nature</span>
<h2 class="text-white font-semibold font-serif text-xl my-5">Spend time with nature to recharge yourself</h2>
<span class="inline-block text-xs text-white font-sans">December 02, 2019. By Debbie</span>
</div>
</div>
</a>
</li>
...
</ul>
</div>
</div>
With this code, we have created a beautiful card layout with all the necessary information for the featured posts.
The classes are very self explanatory like inline-block
, mx-auto
which translate to display: inline-block
and margin: 0 auto
respectively. A basic knowledge of CSS is all you need to get going with tailwindcss. Also, there are code autocomplete plugins/extentions for most code editors.
You get the idea now. I’ll include the entire page code at the end.
Lets dive into some interesting tailwind features.
Custom CSS with tailwindcss
Sometimes you may need custom values to adjust a component to your liking, tailwind provides a configuration option through tailwind.config.js. Tailwind docs provide a neat guide for this. Have a look. You need to be using the npm version of tailwind instead of CDN for this.
<div class="mt-20">
<h2 class="font-light text-xl mb-5 text-center">Search the blog</h2>
<div class="relative border rounded-sm overflow-hidden">
<form action="">
<input class="w-full relative p-5 font-light text-gray-900 border-0" type="text" name="s" id="" placeholder="Search...">
<button type="submit" class="bg-transparent border-0 absolute top-auto right-0 px-5 py-5" style="top: 2px">
<span class="block w-5">
<svg class="fill-current" xmlns="http://www.w3.org/2000/svg" viewBox="0 0 512 512">...</svg>
</span>
</button>
</form>
</div>
</div>
Here, as you can see, I needed a top position 2px adjustment for the search button icon. Instead of writing the needed css in a style attribute as shown above, we can use the tailwind config.
It looks like this. I followed tailwind docs to make the changes to my tailwind.config.js
module.exports = {
theme: {
extend: {
inset: {
'2': '2px',
}
}
},
variants: {},
plugins: []
}
This gives me a new utility class after runing the build command. So now i can use top-2
utility class instead of style="top: 2px"
.
Extraction
As you can see, we have lots of utility classes that we are using and it can become hard to maintain when you have repeated components like we have here in a blog template. Tailwind provides a way to make life easier when dealing with duplication.
This is a repeated component to display recent posts in the page body.
<div class="w-1/2 overflow-hidden pr-2 md:pr-4">
<div>
<img class="w-full h-auto rounded" src="..." alt="">
<h2 class="text-gray-900 font-thin font-serif text-xl my-5"><a href="">...</a></h2>
<p class="text-gray-900 font-thin tracking-wider leading-loose">...</p>
<a href="" class="inline-block pt-5 text-sm font-medium tracking-wider">Read More...</a>
</div>
</div>
We can use tailwind @apply directive to extract these utility classes into CSS component classes. So the code will look something like this:
<div class="article-card">
<div>
<img class="article-image" src="..." alt="">
<h2 class="article-title"><a href="">...</a></h2>
<p class="article-body">...</p>
<a href="" class="readmore">Read More...</a>
</div>
</div>
You should add the new component classes directly after @tailwind components
directive and before @tailwind utilities
directive to avoid specificity issues. You can see what I mean in my tailwind.css file here.
@tailwind base;
@tailwind components;
.article-row {
@apply flex flex-wrap overflow-hidden mb-10;
}
.article-card-left {
@apply w-1/2 overflow-hidden pl-2;
}
.article-card-right {
@apply w-1/2 overflow-hidden pr-2;
}
@screen md {
.article-card-left {
@apply pl-4;
}
.article-card-right {
@apply pr-4;
}
}
.article-image {
@apply w-full h-auto rounded;
}
.article-title {
@apply text-gray-900 font-thin font-serif text-xl my-5;
}
.article-body {
@apply text-gray-900 font-thin tracking-wider leading-loose;
}
.readmore {
@apply inline-block pt-5 text-sm font-medium tracking-wider;
}
@tailwind utilities;
You will notice that for md:pr-4
i have used the plain version of the class in a media query, this is how it works. Tailwindcss docs reads:
It’s important to understand that
@apply
will not work for inlining pseudo-class or responsive variants of another utility. Instead, apply the plain version of that utility into the appropriate pseudo-selector or a new media query.
That is all you need to build a beautiful blog template using tailwindcss.
Get it for Free
I have created the home page, and a few other pages which you can download from here.
Its on Github, you can clone the repo instead of downloading if you like. Here it is.
Enjoy!